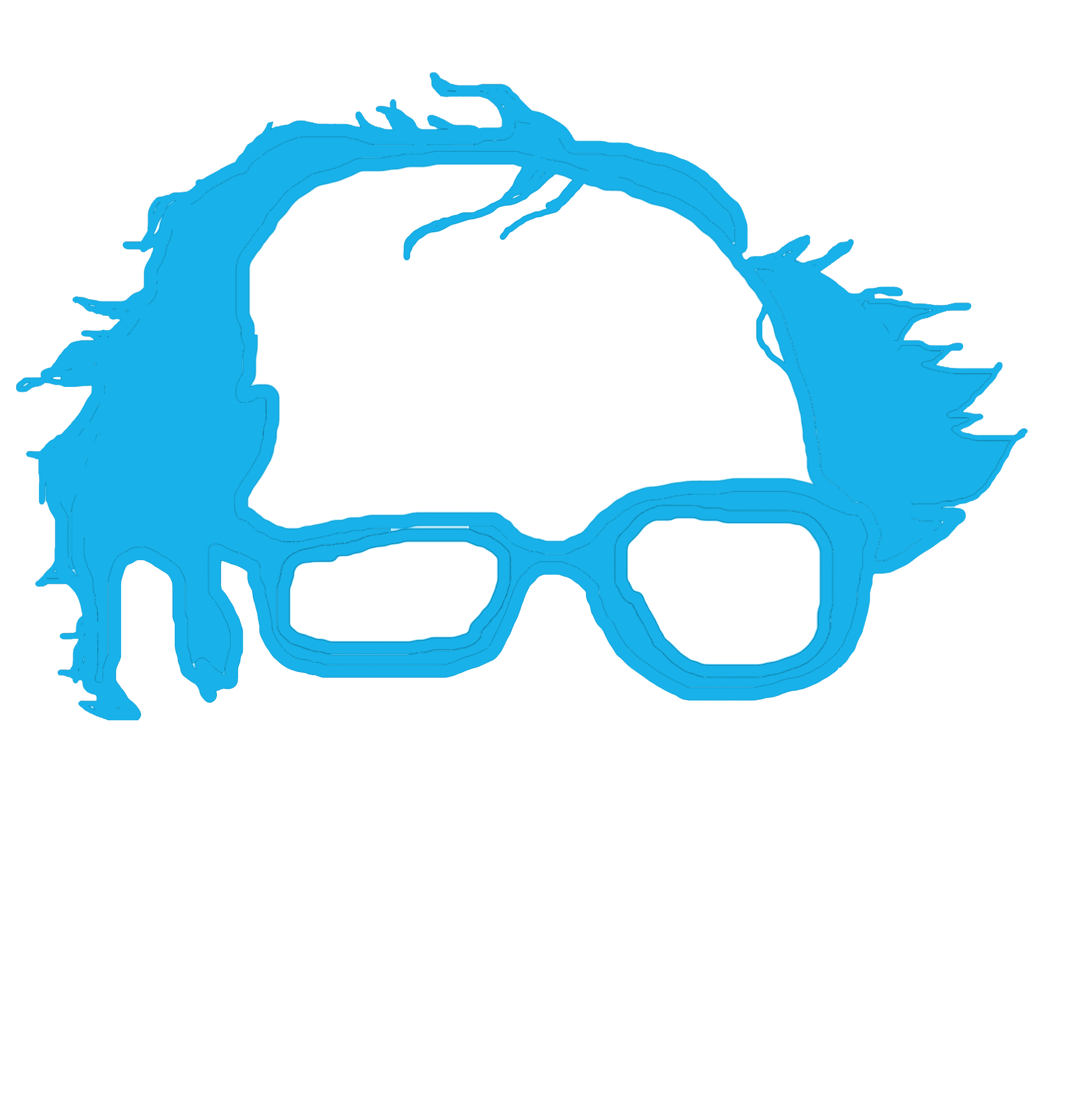
Let’s face it: hashtags on Twitter can be a lot of fun. Not only are they a strangely satisfying way to annoy our friends and followers, but they’ve become a uniquely modern way of categorizing our thoughts. No longer are the days of sifting through every news article and interview; now, just search #feelthebern, and BAM! 140 characters of the latest Bernie information is right at your fingertips.
Hashes in Ruby are actually pretty similar. You can search for a value that is associated with a unique key identifier. If, for example, I created a political hash, I would use the following code:
politics = {
"Bernie" => "basically the coolest ever",
"Clinton" => "standard politician",
"Trump" => "America, what are you thinking."
}
I could then use the Bernie identifier to access my political stance. By typing puts politics["Bernie"]
, I can print out:
basically the coolest ever
Each key in my hash has a unique associated value. Can you guess what will happen if I type puts politics["Trump"]
?
I get the value, America, what are you thinking.
Hashes are great, and super useful if we want to associate objects together. But, what if we want to share our political views with our friends? What if Becky shows up to the polls on voting day, and doesn't know anything about the candidates? Shame, shame Becky.
Well, we could get her access to our politics hash. If she didn't know the names of any of the candidates, she could print out the entire hash and read the results. But poor Becky only has a couple seconds, and doesn't have the time to read through our lengthy candidate descriptions. She just wants to know who the number one candidate is.
Hashes don't actually give us the opportunity to share that information. Hashes are unordered lists of associated pairs. Even if we put our Bernie/description pair in first, it might not actually exist first in our hash. If we want an ordered list, we have to use an array.
An array is an ordered list. We can create a political array like so:
political_array = ["Bernie", "Clinton", "Trump"]
If Becky wanted to know the number one candidate, all she would have to do is access the first item in the array. The code puts political_array[0]
would print Bernie
.
If we really wanted to help Becky out, we could create a 2D array. A 2D array allows us to associate two objects together in an ordered way. If we write the following code:
political_2D_array = [
["Bernie","basically the coolest ever"],
["Clinton","standard politician"],
["Trump", "America, what are you thinking."]
]
Becky could code puts political_2D_array[0]
to get the information at the first index. She would get:
Bernie
basically the coolest ever
If she was feeling curious about why I didn't like the second place candidate, but didn't care enough to know her name, she could type puts political_2D_array[1][1]
to access the information in the second index, at the second position. Similarly, she could do the same with the description in the 3rd index using puts political_2D_array[2][1]
to print out America what are you thinking.
In this way, we can use hashes, arrays, and multidimensional arrays to ourganize our thoughts and code. We can access different values and associated pairs by understanding the differences between ordered and unordered information. And in this way, we can slowly get the world to feel the Bern.