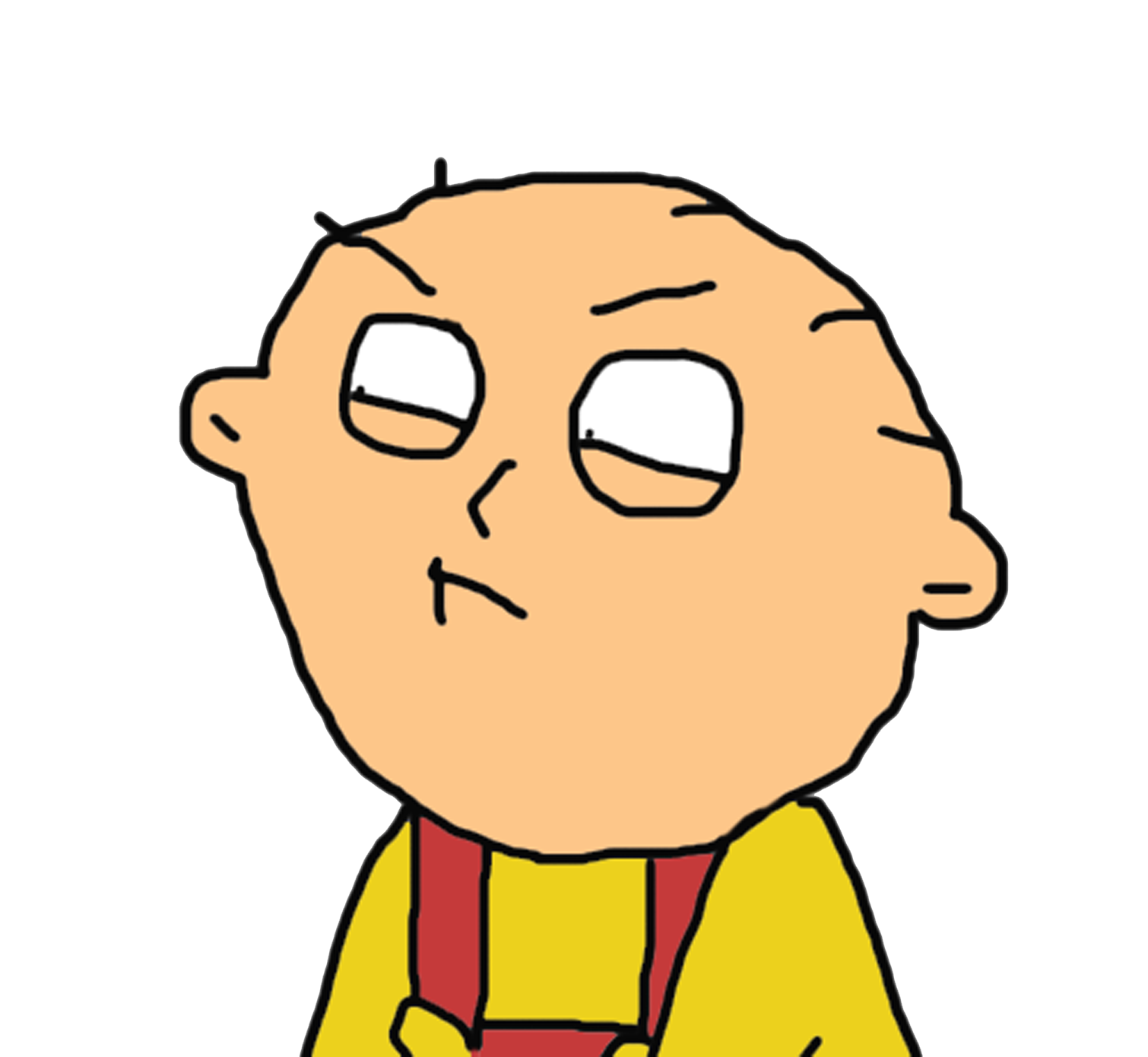
If I'm talking to Paul about how much money he owes me (for the 19th time), I could pull out my handy dandy Money Owed list, and read it off to him.
"Hey Paul. You owe me money. $50 for dinner on Friday, $26 for drinks on Saturday, $80 for doing your homework*, $32 for Tacos on Tuesday, and $43 for margaritas at Taco Tuesday."
*I'm smarter than Paul, and need to make some money. Don't judge.
Going through the items on my list one by one is the same thing as enumerating through them. The Enumerable module in Ruby is one of the coolest, because it allows you to go through each of the items in a collection and do something to them. If, for example, I want to enumerate through my money owed list and print out a hardcopy for Paul, I can do this:
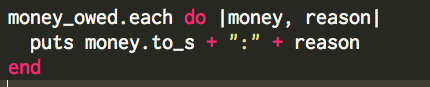

See that .each method that I'm using on my hash? It means that I'm going through each one of the items in my list and puts-ing them out. If you can use the .each method on your list, you can use a method from the enumerable module.
If you want to take a look at all the methods available to you through the enumerable module, take a look at the Ruby Docs. They can be a little overwhelming, so I'll go through a couple that I think will be helpful (aka the ones that will help Paul remember how much money he owes me).
Let's say I wanted to print out everything that Paul owed me that was more than $30. I would have to iterate over each item in the list, and compare it to 30. If the amount owed is more than 30, I want to print it. If not, we won't print it out (sometimes you have to pick your battles with Paul).
We could use the .each method and a for/else statement:
OR, we can be lazy efficient, and use a method from the enumerable module. Let's go with .find_all:
If I wanted to find out how many things Paul owes me for, I can count the size of my list. This is the same as iterating over the entire list, and keeping track of how many items I was iterating over. We can write this like so:
to end up with 5. OR, use the handy .count method from the enumerable module:
to yield the exact same result.
Let's say Paul wants to be on a payment plan. He wants to pay off the smallest sum first. We could iterate over the whole list and compare amounts, OR, you guessed it, use a method from the enumerable module. In this case, .min:
What about if I wanted to group together all the things that Paul owes me for, by the reason he owes me? We can use a handy method: .group_by:
What if Paul only has $10 bills on him, so he contests that he can't start paying me because I don't have any change? Well, let's get him a list of what he can pay me for using just $10 bills:
And finally, let's show Paul how much money he'd owe me if I added 20% interest on each item as a late fee:
And sum it up for him:
There you have it. Paul, give me my money. I've told you now in 11 different ways.